Python Number Formatting Made Easy with f-Strings
Python f-strings are a way to format strings in a concise and readable manner. With f-strings, you can include expressions inside curly braces {} that will be evaluated at runtime and replaced with their values in the resulting string. Formatting numbers with f-strings is also straightforward and offers a lot of flexibility.
There are several formatting options that can be used with f-strings. The most commonly used ones for numbers are:
{variable:format_spec}
: This is the basic syntax for f-strings. Theformat_spec
is a string that specifies how the variable should be formatted.:d
: Format an integer number as a decimal integer.:f
: Format a floating-point number as a fixed-point number.:e
: Format a floating-point number in scientific notation.:g
: Format a floating-point number as either a fixed-point number or in scientific notation, depending on which is more compact.
Below are some examples of how to use f-strings to format numbers.
Format an integer:
age = 25
print(f"I am {age:d} years old.") # output: I am 25 years old.
Format a floating-point number with fixed-point notation:
pi = 3.14159265
print(f"Pi is approximately {pi:.2f}.") # output: Pi is approximately 3.14.
Format a floating-point number with scientific notation:
avogadro = 6.02214076e23
print(f"Avogadro's number is {avogadro:e}.") # output: Avogadro's number is 6.022141e+23.
Format a floating-point number in either fixed-point or scientific notation:
g = 9.81
print(f"The acceleration due to gravity is {g:g} m/s^2.") # output: The acceleration due to gravity is 9.81 m/s^2.
In the examples above, the expressions inside the curly braces {} are evaluated at runtime and replaced with their values formatted according to the specified format_spec
.
You can also use f-strings to format numbers in more complex ways. For example, you can specify the width and alignment of the output, or include a thousands separator. Below are some examples.
Format an integer with leading zeros and a minimum width of 4 characters:
number = 42
print(f"The answer is {number:04d}.") # output: The answer is 0042.
Format a floating-point number with a minimum width of 10 characters and right alignment:
price = 9.99
print(f"The price is ${price:>10.2f}.") # output: The price is $ 9.99.
Format an integer with a thousands separator:
population = 8027959165
print(f"The population is {population:,} people.") # output: The population is 8,027,959,165 people.
In the examples above, the format_spec
includes additional options that specify the minimum width of the output, the alignment, and the use of a thousands separator.
Conclusion
With f-strings, you can easily format numbers in a variety of ways that are both concise and readable. I hope this tutorial has been helpful in understanding how to format numbers in Python.
Featured Merch
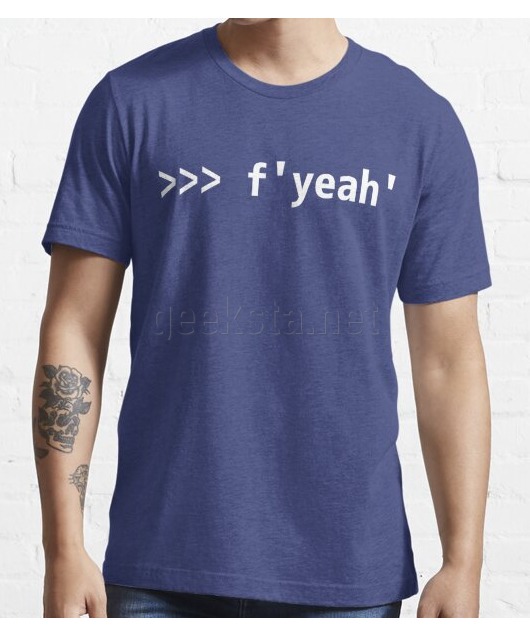
Latest Posts
- Image Prompt Creator: Generate AI Prompts from Images
- Server Failover: A Guide for System Administrators
- Fixing Dovecot Diffie-Hellman Parameter Error
- How to Search and View mbox Email Archives
- Troubleshooting Database Load Issues on Debian Linux: A Practical Guide
Featured Book
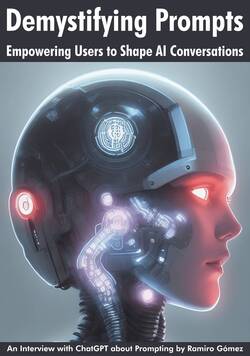
Subscribe to RSS Feed
This post was written by Ramiro Gómez (@yaph) and published on . Subscribe to the Geeksta RSS feed to be informed about new posts.
Tags: code python formatting tutorial
Disclosure: External links on this website may contain affiliate IDs, which means that I earn a commission if you make a purchase using these links. This allows me to offer hopefully valuable content for free while keeping this website sustainable. For more information, please see the disclosure section on the about page.