Drawing the Yin and Yang Symbol with D3.js
The JavaScript code below shows how you can draw the yin and yang symbol using D3.js. After setting the dimensions of the displayed elements and creating the SVG container, we create a clip path to define the visible and hidden portions of the large white circle. This clip path is referenced further below when drawing the large white circle. The rest of the code is drawing the smaller inner circles on top of each other, hence their order is important, to finish the picture.
If you're into Chinese philosophy and like the yin and yang symbol check out the available merchandise products with a negative space yin yang design.
JavaScript Code
const radius = 300,
stroke = 5,
width = (radius + stroke) * 2,
svg = d3.select('#canvas').append('svg')
.attr('width', width)
.attr('height', width);
const g = svg.append('g')
.attr('transform', `translate(${stroke}, ${stroke})`);
g.append('clipPath').attr('id', 'clip').append('rect')
.attr('width', radius)
.attr('height', radius * 2);
// outline
g.append('circle')
.attr('cx', radius)
.attr('cy', radius)
.attr('r', radius)
.attr('stroke', 'black')
.attr('stroke-width', stroke);
// large white circle
g.append('circle')
.attr('cx', radius)
.attr('cy', radius)
.attr('r', radius)
.attr('fill', 'white')
.attr('clip-path', 'url(#clip)');
// inner black circle
g.append('circle')
.attr('cx', radius)
.attr('cy', radius * 1.5)
.attr('r', radius * 0.5);
// white seed
g.append('circle')
.attr('cx', radius)
.attr('cy', radius * 1.5)
.attr('r', radius * 0.15)
.attr('fill', 'white');
// inner white circle
g.append('circle')
.attr('cx', radius)
.attr('cy', radius * 0.5)
.attr('r', radius * 0.5)
.attr('fill', 'white');
// black seed
g.append('circle')
.attr('cx', radius)
.attr('cy', radius * 0.5)
.attr('r', radius * 0.15);
Related Merch
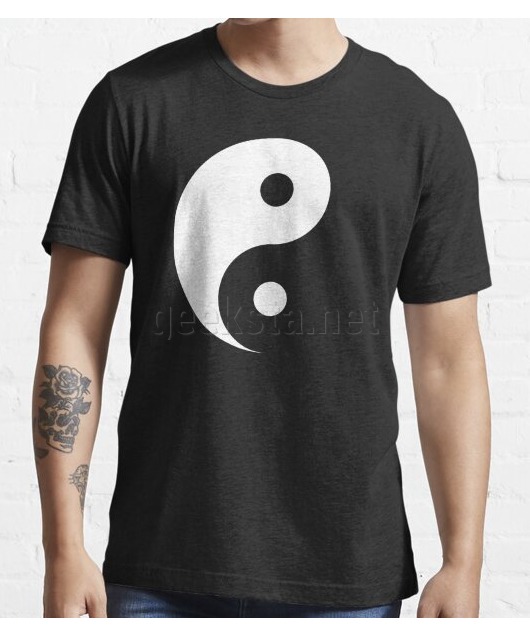
Latest Posts
- Image Prompt Creator: Generate AI Prompts from Images
- Server Failover: A Guide for System Administrators
- Fixing Dovecot Diffie-Hellman Parameter Error
- How to Search and View mbox Email Archives
- Troubleshooting Database Load Issues on Debian Linux: A Practical Guide
Featured Book
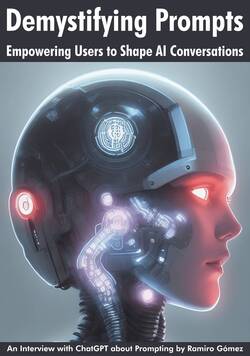
Subscribe to RSS Feed
This post was written by Ramiro Gómez (@yaph) and published on . Subscribe to the Geeksta RSS feed to be informed about new posts.
Disclosure: External links on this website may contain affiliate IDs, which means that I earn a commission if you make a purchase using these links. This allows me to offer hopefully valuable content for free while keeping this website sustainable. For more information, please see the disclosure section on the about page.