Drawing Ramones Style Circular Text with D3.js
The JavaScript code below shows how you can draw circular text as seen in the Ramones logo with SVG paths and SVG text and textPath elements using the D3.js API.
After setting the dimensions of the elements to be displayed, two arcs are defined for drawing two SVG paths. The inner_arc
is not visible but serves as an anchor for the circular texts, that will be attached to this arc's path
element. The outer_arc
displays the white circle enclosing the texts.
The remaining code creates two text
elements, one for the names and one for the stars. The names will be positioned right on the path and the stars will be shifted on the y-axis, so they are rendered inside the invisible inner circle. Finally, the texts are attached/linked to the path using textPath
elements.
Note that the resulting image displays differently in Firefox and Chrome, due to the way they render type. I've set the variables so the image works best in Chrome. In Firefox the circular star text, doesn't quite fit. A possible fix would be to set font sizes based on which browser is being used.
The names are not the first names of the four Ramones, but those of the Authors of the software engineering classic Design Patterns, because I wanted to create a parody design dedicated to the so called Gang of Four.
JavaScript Code
const w = 800,
h = w,
font_scale = w / 8.08,
star_scale = font_scale / 2.1,
stroke_width = (w / 50),
inner_circle = (w / 2) - font_scale,
outer_circle = (w / 2),
fgcolor = '#ffffff',
symbol = '٭',
symbol_count = 50,
text = 'ERICH ★ RICHARD ★ RALPH ★ JOHN ★',
translate = 'translate(' + w / 2 +',' + h / 2 + ')';
const svg = d3.select('#canvas').append('svg')
.attr('width', w)
.attr('height', h)
.attr('style', 'background: #000')
// Create arcs for drawing paths.
const inner_arc = d3.arc()
.innerRadius(inner_circle)
.outerRadius(inner_circle)
.startAngle(0)
.endAngle(360);
const outer_arc = d3.arc()
.innerRadius(outer_circle - stroke_width)
.outerRadius(outer_circle)
.startAngle(0)
.endAngle(360);
// Create paths for aligning texts, and showing the outer circle.
svg.append('path')
.attr('d', inner_arc)
.attr('id', 'inner-circle')
.attr('transform', translate);
svg.append('path')
.attr('d', outer_arc)
.attr('id', 'outer-circle')
.attr('transform', translate)
.attr('fill', fgcolor);
// Create text elements that will be put on the circular paths.
const text_names = svg.append('text')
.attr('x', 0)
.attr('dy', 0)
.style('font-size', font_scale + 'px')
.style('font-family', 'Times New Roman');;
const text_stars = svg.append('text')
.attr('x', 0)
.attr('dy', stroke_width * 2)
.style('font-size', star_scale + 'px');
// Add the first names of the GOF authors.
text_names.append('textPath')
.attr('fill', fgcolor)
.attr('xlink:href','#inner-circle')
.text(text)
// Add the stars below the names.
text_stars.append('textPath')
.attr('fill', fgcolor)
.attr('xlink:href','#inner-circle')
.text(Array(symbol_count).join(symbol + ' '));
Related Merch
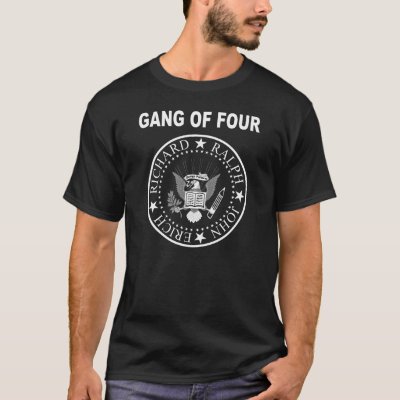
Latest Posts
- Image Prompt Creator: Generate AI Prompts from Images
- Server Failover: A Guide for System Administrators
- Fixing Dovecot Diffie-Hellman Parameter Error
- How to Search and View mbox Email Archives
- Troubleshooting Database Load Issues on Debian Linux: A Practical Guide
Featured Book
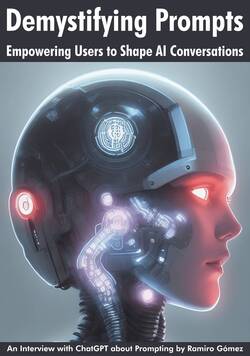
Subscribe to RSS Feed
This post was written by Ramiro Gómez (@yaph) and published on . Subscribe to the Geeksta RSS feed to be informed about new posts.
Disclosure: External links on this website may contain affiliate IDs, which means that I earn a commission if you make a purchase using these links. This allows me to offer hopefully valuable content for free while keeping this website sustainable. For more information, please see the disclosure section on the about page.