How to Import Modules in a Standalone Python Program
When developing a standalone Python application, properly structuring your code and managing imports is key to ensuring your program works consistently—whether you're testing it locally or distributing it with tools like pipx
. In this post, we'll walk through how to design your project, handle imports effectively, and prepare your app for installation.
Why Proper Imports and Project Structure Matter
In Python, how your project is structured significantly impacts module discovery and usability. Mismanaging imports can lead to frustrating ModuleNotFoundError
issues, especially when transitioning between development and production. A clean structure makes your code easier to maintain, test, and distribute.
Project Structure
Let's start by establishing a clear project layout. For this example, suppose you're creating an application called myapp
. Here's our example directory structure:
myapp/
mymodule/
__init__.py
util.py
cli.py
setup.py
Key Elements
mymodule/
: This is the main package containing your application's logic.__init__.py
: Marks the directory as a Python package. Even though Python 3.3+ doesn't require it, adding this file is a good practice, especially for compatibility and clarity.setup.py
: Defines the metadata and entry points for your application, enabling installation and usage as a standalone command.
Absolute vs. Relative Imports
When writing modular code, you'll frequently need to import one part of your application into another. Python supports both absolute and relative imports. Here, we recommend using absolute imports, as they are more explicit and easier to understand.
Example: Using an Absolute Import in cli.py
Suppose cli.py
needs to use a function from util.py
. Here's how you would handle the import:
# mymodule/cli.py
from mymodule import util
def main():
# Your application's entry point
print(util.some_function())
This approach directly specifies where util
resides within the overall package structure, reducing ambiguity.
Running Your Script Locally
To ensure your script runs correctly during development, always execute it with the -m
flag from the root directory of your project. For example:
cd myapp
python -m mymodule.cli
The -m
flag tells Python to treat mymodule.cli
as a module in the package context, making imports like from mymodule import util
work seamlessly.
Important: Avoid Running Modules Directly
Running files directly (e.g., python mymodule/cli.py
) can lead to import errors because Python won't treat mymodule
as part of a package. Always use the -m
approach for package-based scripts.
Preparing for Installation with pipx
Distributing your application as a standalone tool is simple with pipx. First, you need to create a setup.py
file to define your package and its entry point.
Example: setup.py
Here's a minimal setup.py
file:
from setuptools import setup, find_packages
setup(
name='myapp',
version='0.1',
packages=find_packages(),
entry_points={
'console_scripts': [
'myapp=mymodule.cli:main',
],
},
)
What's Happening Here?
find_packages()
: Automatically detects all your Python packages, including sub-packages likemymodule
.entry_points
: Defines a command-line executable (myapp
) that maps to themain()
function inmymodule.cli
.
Configuring the Entry Point
Your CLI script (cli.py
) needs a main()
function to be callable from the command line. Here's how it should look:
# mymodule/cli.py
from mymodule import util
def main():
# Your application logic goes here
print(util.some_function())
if __name__ == "__main__":
main()
The main()
function is included in the setup.py
file as the starting point of your application.
Testing and Installing with pipx
Once your project is ready, you can use pipx to install and test it:
- Navigate to the root of your project directory:
cd myapp
- Install the application with pipx:
pipx install .
- Run your application from anywhere using the executable name defined in your
setup.py
(myapp
in this case):
myapp
By relying on pipx, your app will work in an isolated environment, reducing the need to manage Python dependencies globally.
Conclusion
Managing imports and structuring your project correctly paves the way for both smooth development and seamless distribution. Using absolute imports helps maintain clarity and consistency across your codebase, while tools like pipx make installing and running standalone applications a breeze.
By following the steps outlined here, you'll be well-equipped to create polished, professional Python applications that are easy to share and use. If you plan to publish your app to PyPI, consider adding a pyproject.toml
file for simplified dependency and build management. Happy coding! 🐍
Featured Merch
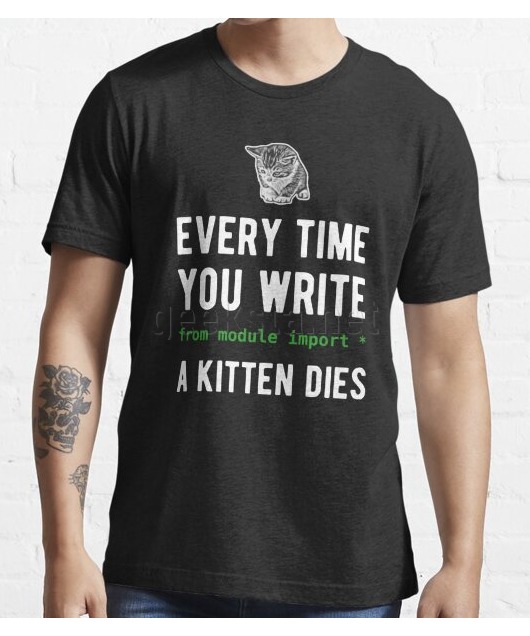
Latest Posts
- How to Use SD Cards for Backups on Linux
- Wiki Story: Turn Wikipedia Articles into Captivating Narratives
- How to Determine the SMTP and IMAP Servers for a Domain
- How to Determine the Email Server (MX Records) for a Domain
- How to Set max_input_vars in PHP: A Quick Guide for Developers
Featured Book
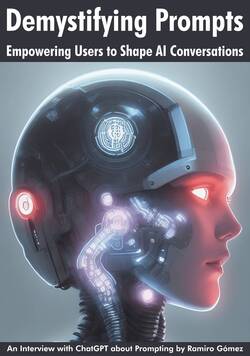
Subscribe to RSS Feed
This post was written by Ramiro Gómez (@yaph) and published on . Subscribe to the Geeksta RSS feed to be informed about new posts.
Tags: howto pipx python software development
Disclosure: External links on this website may contain affiliate IDs, which means that I earn a commission if you make a purchase using these links. This allows me to offer hopefully valuable content for free while keeping this website sustainable. For more information, please see the disclosure section on the about page.