How to Exit a Python Program Gracefully
When developing Python programs, it's crucial to exit gracefully — whether your program ends by design or due to unexpected interruptions. Graceful exits ensure that resources are released, cleanup tasks are completed, and users receive clear feedback.
In this guide, we'll explore the most common ways to exit a Python program cleanly with practical examples and best practices.
1. Using sys.exit()
The most reliable, built-in way to terminate a Python program in scripts or production environments is sys.exit()
from the sys
module. It allows you to stop program execution immediately and optionally send a message or return an exit code for external tools or systems.
Example:
import sys
def main():
print("This is the main part of the program.")
# Perform your program tasks here
print("Now quitting the program.")
# Exit with a message
sys.exit("Goodbye! The program is now exiting.")
if __name__ == "__main__":
main()
Explanation:
- The
sys.exit()
function stops the entire program. - If provided, the argument (e.g.,
"Goodbye!"
) is displayed to the console, or passed to the parent process when used in automation pipelines.
Tip: Use an integer (0 for success, non-zero for errors) if your program is part of a pipeline or being managed/testing by external tools, such as shell scripts or CI/CD systems.
2. Handling Keyboard Interrupts (Ctrl+C
)
When users terminate your program by pressing Ctrl+C
, Python raises a KeyboardInterrupt
exception. It's a good practice to handle this exception explicitly, ensuring the program exits cleanly and provides feedback.
Example:
import sys
def main():
try:
print("Program is running. Press Ctrl+C to exit.")
# Simulate a long-running task
while True:
pass # Replace with actual logic
except KeyboardInterrupt:
print("KeyboardInterrupt detected. Cleaning up and exiting...")
sys.exit(0) # Exit gracefully with exit status 0
if __name__ == "__main__":
main()
Why Handle KeyboardInterrupt
?
- Failing to catch
KeyboardInterrupt
might leave critical cleanup tasks incomplete (e.g., closing files, shutting down threads, or releasing database locks). - Adding a friendly exit message improves the user experience for interactive programs.
Tip: Replace infinite loops with specific long-running tasks in production for better program reliability.
3. Understanding the sys.exit()
Function Signature
The signature for sys.exit()
provides flexibility in how you terminate your program:
sys.exit([arg])
The optional arg
parameter controls the exit behavior:
- Integer (recommended):
0
indicates successful termination, while non-zero integers (e.g.,1
) indicate an error or abnormal termination.sys.exit(1)
signals an error occurred. - String: If a string or other object is passed, it will be printed to
stderr
, and an exit status of1
is returned:sys.exit("An error occurred.")
- None: Defaults to
0
(successful termination):sys.exit()
Tip: Use numeric exit codes with external-facing applications, especially if they interact with other services or scripts. Reserve strings for user-facing messages.
4. Why Avoid Using quit()
and exit()
in Scripts?
Python provides two additional commands, quit()
and exit()
, for exiting programs, but these are intended for use in interactive environments like the Python REPL.
Example (Interactive Mode Only):
>>> quit()
>>> exit()
Why Not Use Them in Programs?
- Designed for REPL:
quit()
andexit()
are part of thesite
module, not the Python core. They don't work well in all environments, especially when running scripts or within larger systems. - Inconsistencies: In non-interactive environments (e.g., automation scripts or server applications), they often fail to terminate programs reliably.
Best Practice: Always use sys.exit()
in scripts and production code. Reserve quit()
and exit()
for ad-hoc testing or experimenting in the REPL.
5. Avoiding Abrupt Exits with os._exit()
The os._exit()
function exists for extreme cases where you need to terminate a program immediately — bypassing normal cleanup processes like flushing I/O buffers or garbage collection. This is rarely advisable.
Example:
import os
os._exit(1) # Use only in rare circumstances
When to Use:
- Reserved for advanced scenarios such as terminating worker processes in multithreaded systems.
- Avoid in most cases, as it skips important teardown steps, potentially leaving resources in an unstable state.
Tip: Stick to sys.exit()
unless you absolutely need the low-level control provided by os._exit()
.
6. Final Tips for Exiting Gracefully
- Release Resources: Always ensure files, sockets, or database connections are properly closed before exiting. Use context managers wherever possible.
with open("example.txt", "r") as file:
data = file.read()
# File automatically closed on exit
- Provide Feedback: Show meaningful messages when exiting due to errors or user actions.
- Avoid Silent Failures: Use logging or clear
sys.exit()
exit codes when terminating during errors.
Conclusion
Graceful program termination is a small but essential aspect of Python programming. By using sys.exit()
where appropriate, handling KeyboardInterrupt
exceptions, and avoiding improper approaches like quit()
or os._exit()
in production code, you can ensure clean and predictable program exits.
With these best practices, you'll not only improve your code's reliability but also enhance the user experience for anyone running your Python programs.
Featured Merch
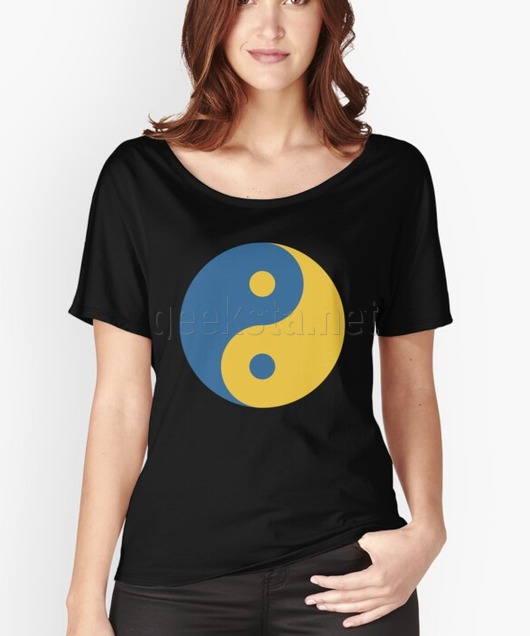
Latest Posts
- How to Use SD Cards for Backups on Linux
- Wiki Story: Turn Wikipedia Articles into Captivating Narratives
- How to Determine the SMTP and IMAP Servers for a Domain
- How to Determine the Email Server (MX Records) for a Domain
- How to Set max_input_vars in PHP: A Quick Guide for Developers
Featured Book
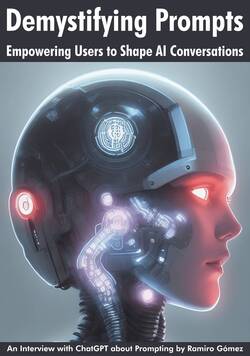
Subscribe to RSS Feed
This post was written by Ramiro Gómez (@yaph) and published on . Subscribe to the Geeksta RSS feed to be informed about new posts.
Tags: howto python software development
Disclosure: External links on this website may contain affiliate IDs, which means that I earn a commission if you make a purchase using these links. This allows me to offer hopefully valuable content for free while keeping this website sustainable. For more information, please see the disclosure section on the about page.