Python's Global Interpreter Lock (GIL): Understanding the Pros and Cons
Introduction
As a Python developer, one topic that frequently sparks debates and controversies is the Global Interpreter Lock, commonly known as the GIL. The GIL is a mechanism present in the CPython interpreter, the default and most widely used implementation of Python.
In simple terms, the GIL is a mutex that allows only one thread to execute Python bytecode at a time, regardless of the number of CPU cores available. This means that in multi-threaded Python applications, only one thread can execute Python code at any given moment, while others have to wait their turn. Consequently, the GIL has both proponents and critics due to its impact on Python's performance and concurrency model.
In this article, I will discuss the pros and cons of Python's Global Interpreter Lock, shedding light on the trade-offs it presents and how it influences Python's suitability for different types of applications. Let's explore the benefits and limitations of the GIL to understand its role in Python's programming landscape.
The Pros of Python's GIL
Simplicity and Stability
The GIL's primary advantage lies in the simplicity it brings to the implementation of CPython, the reference implementation of Python. By allowing only one thread to execute Python bytecode at a time, CPython avoids the complexities of managing fine-grained locks around Python objects. This design choice contributes to CPython's stability and maturity, making it a reliable option for various applications.
Moreover, the GIL ensures thread safety for C extensions used in Python. Since only one thread can execute Python bytecode at any given moment, C extensions do not need to implement intricate synchronization mechanisms for thread safety. This simplifies the development process when integrating Python with existing C or C++ libraries, as developers can interact with Python objects from C/C++ code without worrying about concurrency issues.
Easier Integration with C/C++ Code
The GIL facilitates seamless integration between Python and C/C++ codebases. With the GIL in place, Python developers can effortlessly interact with C/C++ libraries, leveraging their performance and functionality within Python applications. This interoperation is especially beneficial for projects that rely on existing C/C++ codebases or high-performance computational tasks, as it allows Python to tap into the vast ecosystem of C/C++ libraries.
Furthermore, the GIL simplifies memory management in CPython. Since only one thread can execute Python bytecode at a time, memory management operations, such as garbage collection, can be performed without the need for complex synchronization mechanisms. This reduces the risk of memory-related bugs that might arise due to concurrent memory access in multi-threaded applications.
Efficiency in I/O-Bound Operations
While the GIL has performance limitations for CPU-bound tasks, it excels in scenarios where the application's bottleneck is I/O-bound. In I/O-bound operations, the performance is primarily determined by external factors such as network latency, disk I/O, or user input. Since the GIL releases when threads perform I/O operations, it allows other threads to execute during these waiting periods, ensuring that the application remains responsive and efficient in handling I/O-bound tasks.
Summary
Python's Global Interpreter Lock provides simplicity, stability, and thread safety benefits for CPython, making it a strong choice for integrating with C/C++ libraries and simplifying memory management. Additionally, the GIL showcases its efficiency when tackling I/O-bound tasks, allowing Python applications to handle I/O operations with ease and responsiveness. However, it is essential to explore the other side of the coin, as the GIL also introduces some limitations that impact Python's performance and concurrency in CPU-bound tasks.
The Cons of Python's GIL
Performance Limitations
One of the most significant drawbacks of the GIL is its impact on CPU-bound tasks. In CPU-bound operations, where the performance bottleneck arises from intensive computation, the GIL becomes a limiting factor. Since only one thread can execute Python bytecode at a time, multi-threading benefits are limited on multi-core systems. Even if the application has multiple threads, only one thread can utilize a CPU core at any given moment, leaving the other cores underutilized. This leads to suboptimal CPU utilization and reduced performance for CPU-bound tasks in multi-threaded Python applications.
Concurrency Bottleneck
As the number of threads with substantial Python code execution increases in a multi-threaded application, the GIL can turn into a bottleneck. If a thread spends a significant amount of time executing Python code without releasing the GIL, it hampers the ability of other threads to run concurrently. This phenomenon can result in reduced throughput and responsiveness, limiting the application's overall performance.
Moreover, heavily multi-threaded applications might encounter scalability issues. As the number of threads grows, contention for the GIL increases, leading to more frequent situations where threads need to wait their turn. Consequently, this reduces the potential gains from adding more threads to the application, ultimately limiting the scalability of the application's multi-threading approach.
Difficulty in Writing Thread-Safe Code
The presence of the GIL introduces challenges for developers when writing thread-safe Python code. While the GIL ensures that C extensions are thread-safe, Python code itself is subject to the GIL and can't be executed concurrently by multiple threads. This makes it challenging to achieve true parallelism for CPU-bound tasks within Python.
Summary
Python's Global Interpreter Lock introduces performance limitations for CPU-bound tasks and can become a bottleneck in multi-threaded applications with significant Python code execution. The GIL's presence also presents challenges in writing thread-safe Python code and may require developers to explore alternative concurrency models like multi-processing or asynchronous programming to fully leverage the capabilities of modern hardware.
Mitigation Strategies and Alternatives
To overcome the limitations of Python's Global Interpreter Lock and achieve better concurrency and parallelism, developers can employ various mitigation strategies and alternatives:
Multi-Processing
One effective strategy is to use the multi-processing approach instead of multi-threading. Unlike threads, processes have separate memory spaces and do not share the GIL. By utilizing the multiprocessing module in Python, developers can create multiple processes, each running an independent Python interpreter. These processes can then communicate with each other using inter-process communication mechanisms like pipes or queues. Multi-processing allows true parallelism, enabling each process to utilize a separate CPU core efficiently, making it ideal for CPU-bound tasks.
Asynchronous Programming
Asynchronous programming, using libraries like asyncio, is an alternative concurrency model that doesn't rely on threads or the GIL. Instead, it leverages a single thread to handle multiple I/O-bound tasks concurrently. When a task encounters a blocking I/O operation, the event loop switches to other tasks, maximizing CPU utilization. This approach is well-suited for I/O-bound tasks, where the GIL's limitations are less relevant, and applications can handle multiple I/O operations efficiently.
External Libraries in GIL-Free Languages
For CPU-bound tasks that heavily rely on parallel processing, developers can offload the critical parts of their code to external libraries written in GIL-free languages like C, C++, or Rust. These languages do not have a GIL and can efficiently leverage multi-core systems for CPU-bound computations. Python allows easy integration with such external libraries using various tools like ctypes, CFFI, or Cython, enabling developers to harness the power of GIL-free languages within their Python applications.
Using Different Python Implementations
Python has multiple implementations, and some of them do not have a GIL. For instance, PyPy is a popular alternative implementation of Python that uses a Just-in-Time (JIT) compiler, providing performance improvements and automatic memory management without the GIL. Depending on the nature of the application, switching to a GIL-free implementation like PyPy might offer better performance for CPU-bound tasks.
Load Balancing and Task Distribution
In scenarios where multi-threading is necessary, developers can implement load balancing and task distribution techniques to minimize contention for the GIL. By efficiently distributing tasks among threads or processes, developers can reduce the likelihood of threads being blocked by the GIL, thus improving overall performance.
Conclusion
In this article, we have explored the pros and cons of Python's Global Interpreter Lock and its impact on Python's performance and concurrency model. Let's summarize the key points:
The Pros of Python's GIL:
- The GIL simplifies the implementation of CPython, contributing to its stability and maturity.
- It ensures thread safety for C extensions, making integration with C/C++ code more straightforward.
- Python's GIL facilitates seamless interaction with existing C/C++ libraries and simplifies memory management, reducing memory-related bugs.
- The GIL excels in handling I/O-bound tasks, allowing Python applications to remain responsive and efficient when dealing with external factors like network latency or disk I/O.
The Cons of Python's GIL:
- The GIL imposes performance limitations for CPU-bound tasks, hindering multi-threading benefits on multi-core systems.
- It can become a bottleneck in multi-threaded applications with significant Python code execution, leading to reduced throughput and scalability issues.
- Writing thread-safe Python code under the GIL can be challenging, as true parallelism for CPU-bound tasks within Python is not possible.
Considering these factors, the choice between using Python's GIL or exploring alternative implementations depends on the specific requirements and use case of the application.
If the application primarily involves I/O-bound tasks or extensively relies on existing C/C++ libraries, Python's GIL might not significantly impact performance. In such cases, developers can take advantage of Python's simplicity, stability, and ease of integration with external code.
However, for CPU-bound tasks that demand parallel processing and efficient CPU utilization, exploring alternatives such as multi-processing or asynchronous programming can yield better performance. Additionally, leveraging external GIL-free libraries or considering alternative Python implementations like PyPy can offer enhanced parallelism and resource utilization.
Ultimately, the decision to embrace or work around Python's GIL comes down to a careful consideration of the application's specific requirements, the type of tasks it needs to perform, and the desired balance between simplicity and performance. By understanding the trade-offs and employing appropriate concurrency strategies, developers can make informed choices to optimize their Python applications and achieve the best possible outcomes.
Further Resources:
If you want to dive deeper into Python's Global Interpreter Lock, check out the following articles:
Featured eBooks
Latest Posts
- How to Examine a Remote Linux Server via SSH: A Sysadmin's Guide.
- How to Escape Colons in CSS Classes in JavaScript
- Profitable Freelance Writing: Top Niches & Success Advice
- Unlocking the Potential of Podcasting as a Profitable Online Venture
Featured Merch
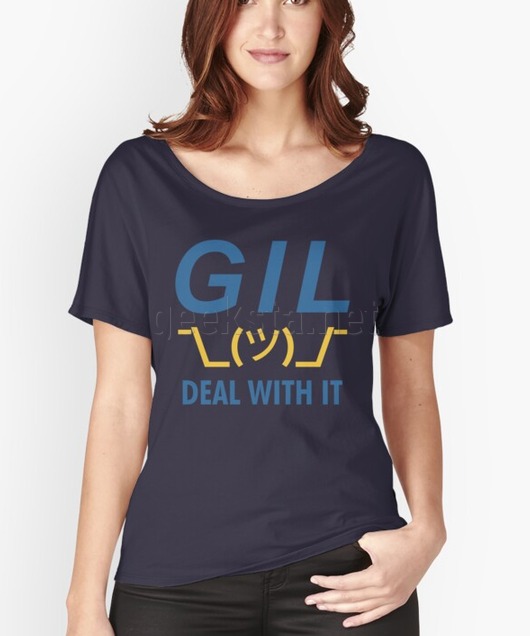
Subscribe to RSS Feed
This post was written by Ramiro Gómez (@yaph) and published on . Subscribe to the Geeksta RSS feed to be informed about new posts.
Tags: python
Disclosure: External links on this website may contain affiliate IDs, which means that I earn a commission if you make a purchase using these links. This allows me to offer hopefully valuable content for free while keeping this website sustainable. For more information, please see the disclosure section on the about page.